What are Config Server and Config Client?
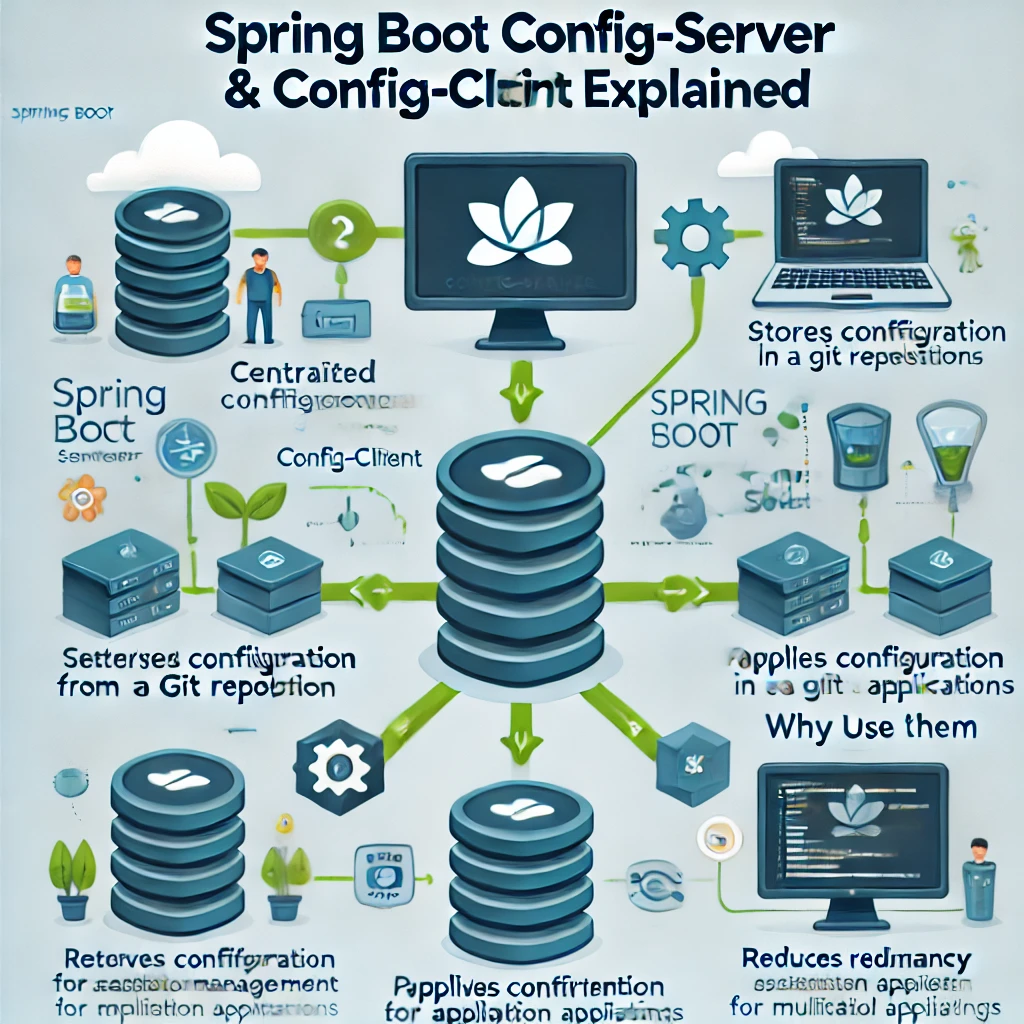
A config server is a central service that provides configuration files for multiple applications. It allows configurations to be managed centrally and changes to be rolled out easily. This is particularly helpful in a microservices architecture, as all services can access the same configuration source.
A config client is an application (one of several microservices) that retrieves its configuration values from the config server. Instead of saving configuration values in each application individually, the client retrieves the required settings dynamically from the server. This reduces redundancy and ensures a consistent configuration across all services.
Why are they necessary?
- Centralized management of configuration files
- Easy rollout of changes without manually customizing each microservice
- Ability to handle environment dependencies dynamically (e.g. different values for development, test and production)
- Improved security by storing sensitive data in a secure location
1. Set up the config server
The config server is the central control center for all configuration files of your microservices. Let’s get started!
Create project
First, we need a new Spring Boot 3 project with the following dependencies:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-server</artifactId>
</dependency>
Server configuration
Create a new class and annotate it with @EnableConfigServer:
@SpringBootApplication
@EnableConfigServer
public class ConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigServerApplication.class, args);
}
}
Configure in bootstrap.yml / application.yml
The server needs a source for the configuration files. A Git repository is usually used. Here is an example:
server:
port: 8888
spring:
cloud:
config:
server:
git:
uri: https://github.com/your-user/your-config-repo
Now start your config server and you can retrieve the configuration files, e.g. with http://localhost:8888/dein-service/default
2. Set up Config Client
Now we need a client that pulls the configuration from the server.
Create project
Here we need the following dependency:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
Configure in bootstrap.yml / application.yml
The client must know where the Config server is located:
spring:
application:
name: your-service
cloud:
config:
uri: http://localhost:8888
Access to configuration values
Now you can retrieve values from the config server with @Value or @ConfigurationProperties:
@RestController
public class ConfigTestController {
@Value("${custom.property:Not found}")
private String customProperty;
@GetMapping("/config")
public String getConfigValue() {
return "Configuration value: " + customProperty;
}
}
3. Apply changes to the configuration
If you change a configuration in your Git repo, your client must be aware of this. This can be done with a refresh endpoint:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Activate the refresh feature:
management:
endpoints:
web:
exposure:
include: refresh
Now you can initiate a configuration change with the following command:
curl -X POST http://localhost:8080/actuator/refresh
Fazit
The central management of configurations with Spring Boot 3 and the Spring Cloud Config Server makes the maintenance and scaling of applications much easier. But be careful: even small errors in the configuration can cause vulnerabilities and thus be a gateway for attackers. Misconfigurations can lead to sensitive data remaining unprotected or systems becoming unexpectedly vulnerable. Especially in distributed systems, it is essential to keep configurations secure and check them regularly.
If you need support in securing your applications, you will find experienced security experts on the cyberphinix marketplace who can help you identify and eliminate potential risks. Protect your systems proactively before vulnerabilities are exploited!