Spring Boot Actuator is one of those features that developers either love or ignore – until they really need it. It provides valuable insights into the state of an application and helps with monitoring and troubleshooting. In this article, we look at how to use Spring Boot Actuator effectively, which endpoints are important and how to configure it optimally in practice.
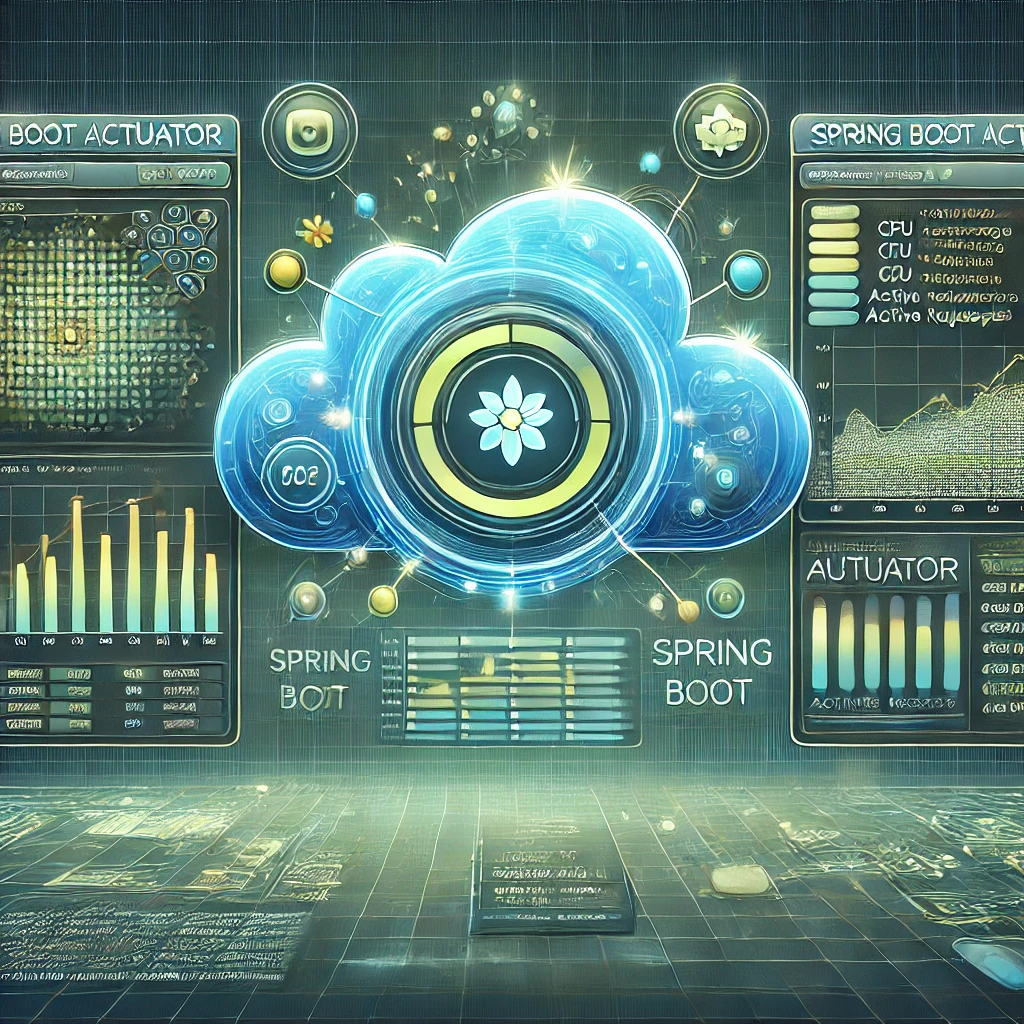
1. What is Spring Boot Actuator?
Spring Boot Actuator is a module of Spring Boot that provides a set of production and monitoring functions for an application. It enables the collection of metrics, health checks and detailed insights into the runtime environment.
Why is Actuator useful?
- Enables health checks to determine whether the application is still working properly.
- Provides metrics to monitor performance and usage statistics.
- Supports tracing and logging to better analyze errors.
- Integrates with Prometheus, Grafana and other monitoring tools.
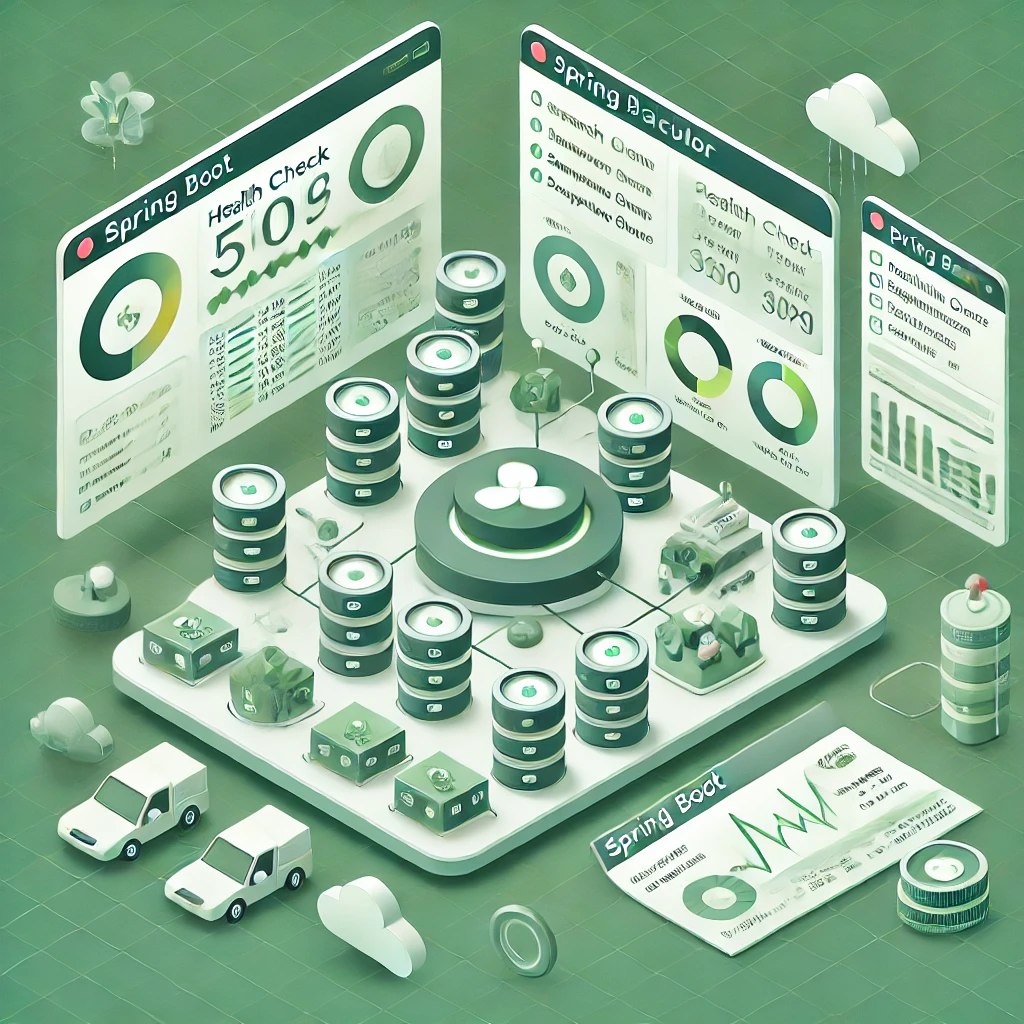
2. Activate Actuator in Spring Boot
To use Actuator in a Spring Boot application, you only need to add the dependency in the pom.xml:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Alternatively for Gradle:
implementation 'org.springframework.boot:spring-boot-starter-actuator'
After restarting the application, the actuator endpoints are available.
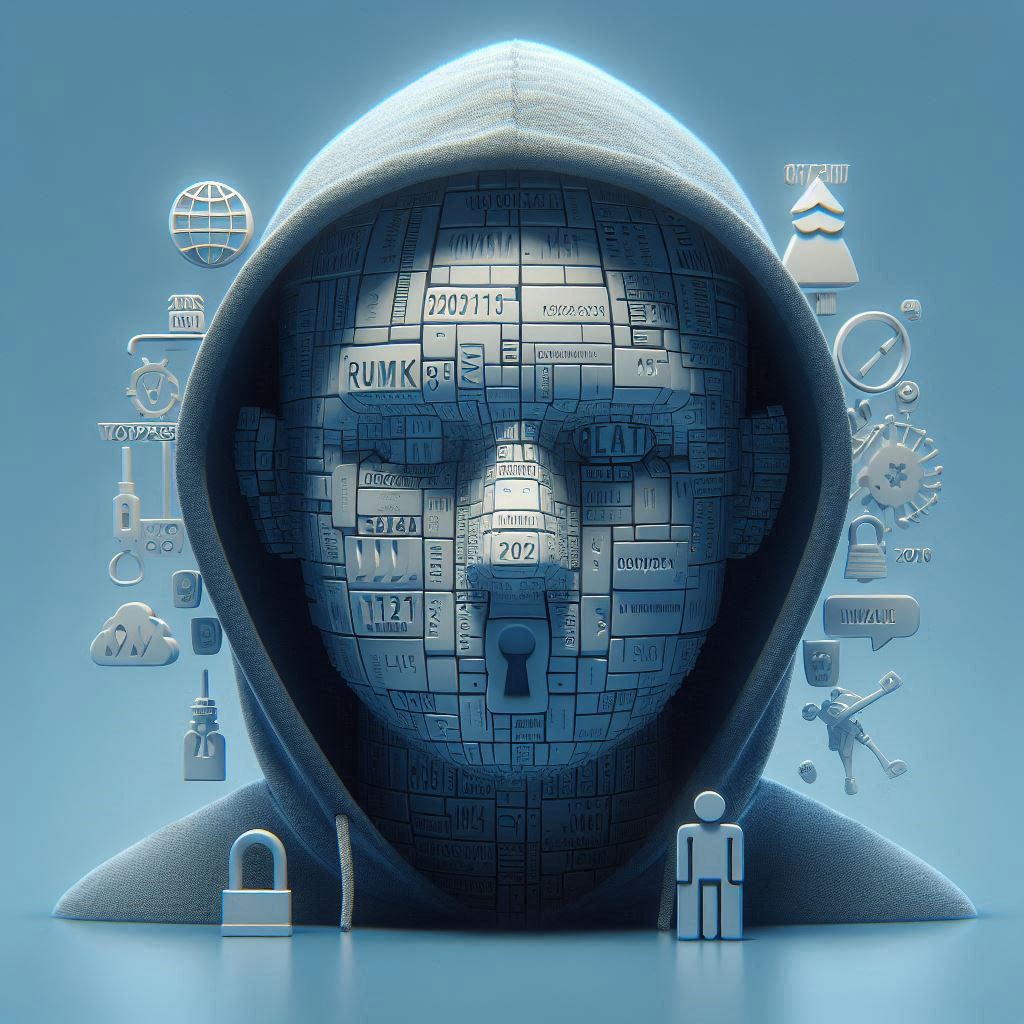
3. Important actuator endpoints
Spring Boot Actuator offers a variety of endpoints that are accessible via HTTP. Here are the most important ones:
Endpoint | Description |
/actuator/health | Displays the health status of the application. |
/actuator/info | Shows general information about the application. |
/actuator/metrics | Provides various metrics. |
/actuator/loggers | Enables the log level to be changed at runtime. |
/actuator/env | Shows the currently loaded environment variables. |
/actuator/beans | Lists all beans in the Spring context. |
Example: Health check
By default, /actuator/health provides a simple status message:
{
"status": "UP"
}
The output becomes more detailed with additional dependencies (e.g. database connection):
{
"status": "UP",
"components": {
"db": { "status": "UP", "details": { "database": "MySQL", "version": "8.0.29" } },
"diskSpace": { "status": "UP", "details": { "total": 500107862016, "free": 314573959168 } }
}
}
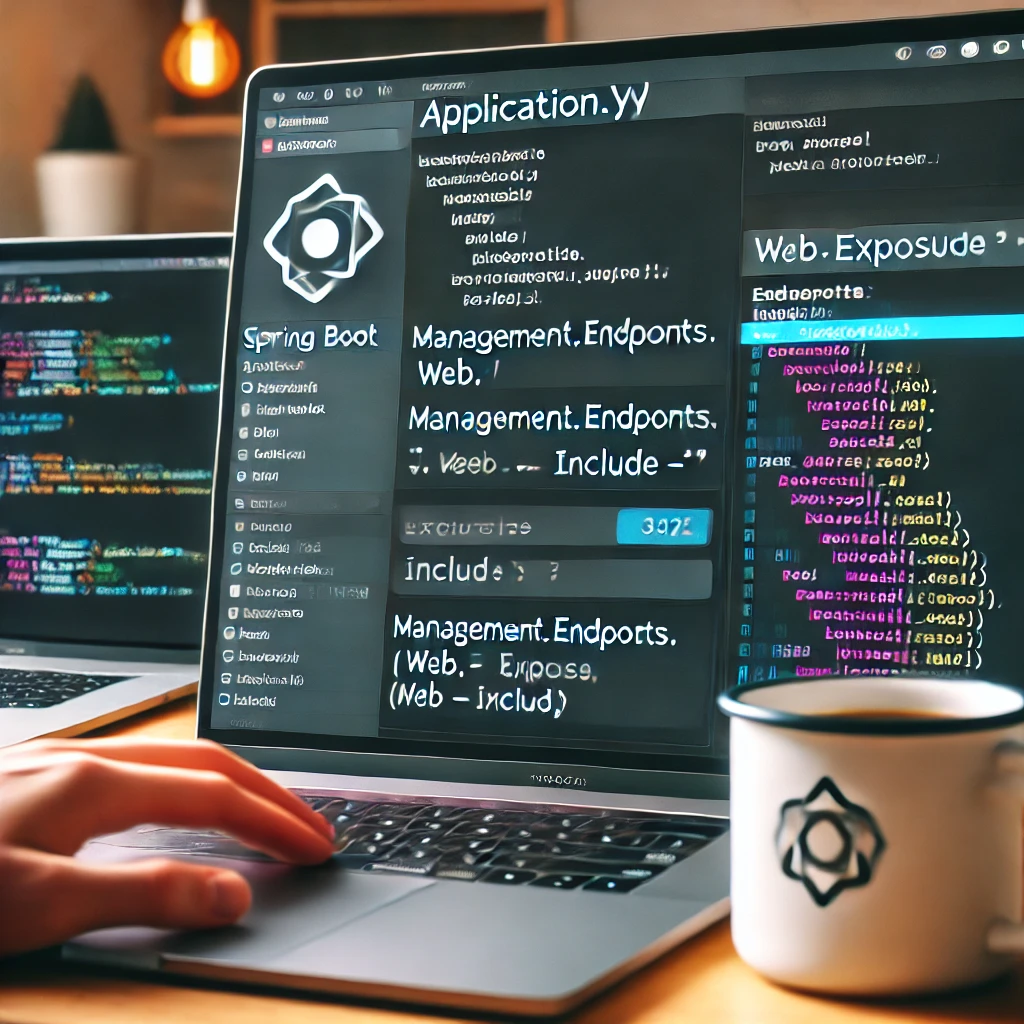
4. Configure actuator endpoints
By default, only a few endpoints are publicly accessible. To enable more, the application.properties or application.yml must be adapted.
Explicitly activate endpoints
management.endpoints.web.exposure.include=health,info,metrics,loggers
Alternatively, to activate all end points:
management.endpoints.web.exposure.include=*
Caution: This should only be done in development environments, as sensitive data could be included.
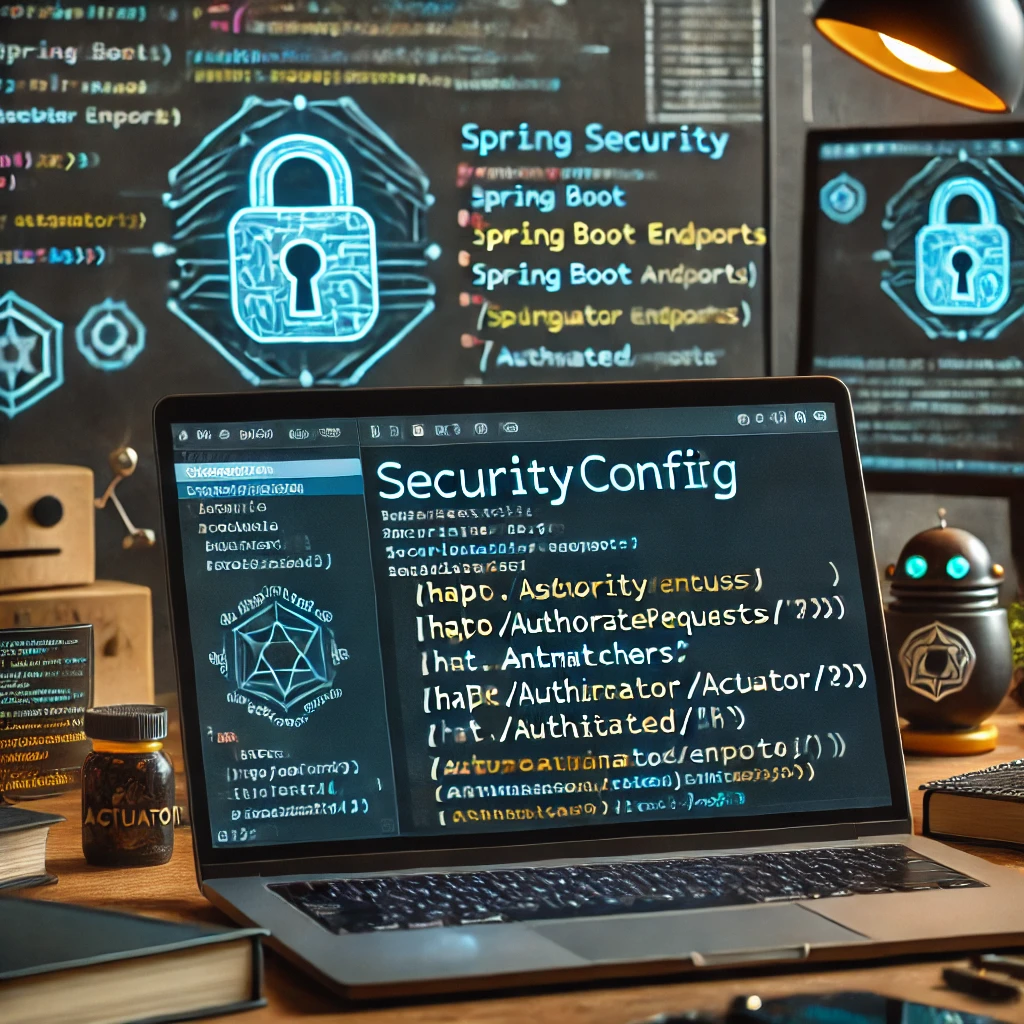
5. Security for actuator endpoints
As Actuator provides detailed insights into the application, access should be secured. Spring Security can be used to provide endpoints with authentication.
Activate Basic Auth for Actuator
In the application.properties:
spring.security.user.name=admin
spring.security.user.password=secrepassword
Now the Actuator endpoints can only be accessed with these credentials.
Alternatively, Spring Security can be configured with role-based access control:
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
return http
.authorizeHttpRequests(auth -> auth
.requestMatchers("/actuator/**").hasRole("ADMIN")
.anyRequest().authenticated())
.httpBasic(withDefaults())
.build();
}
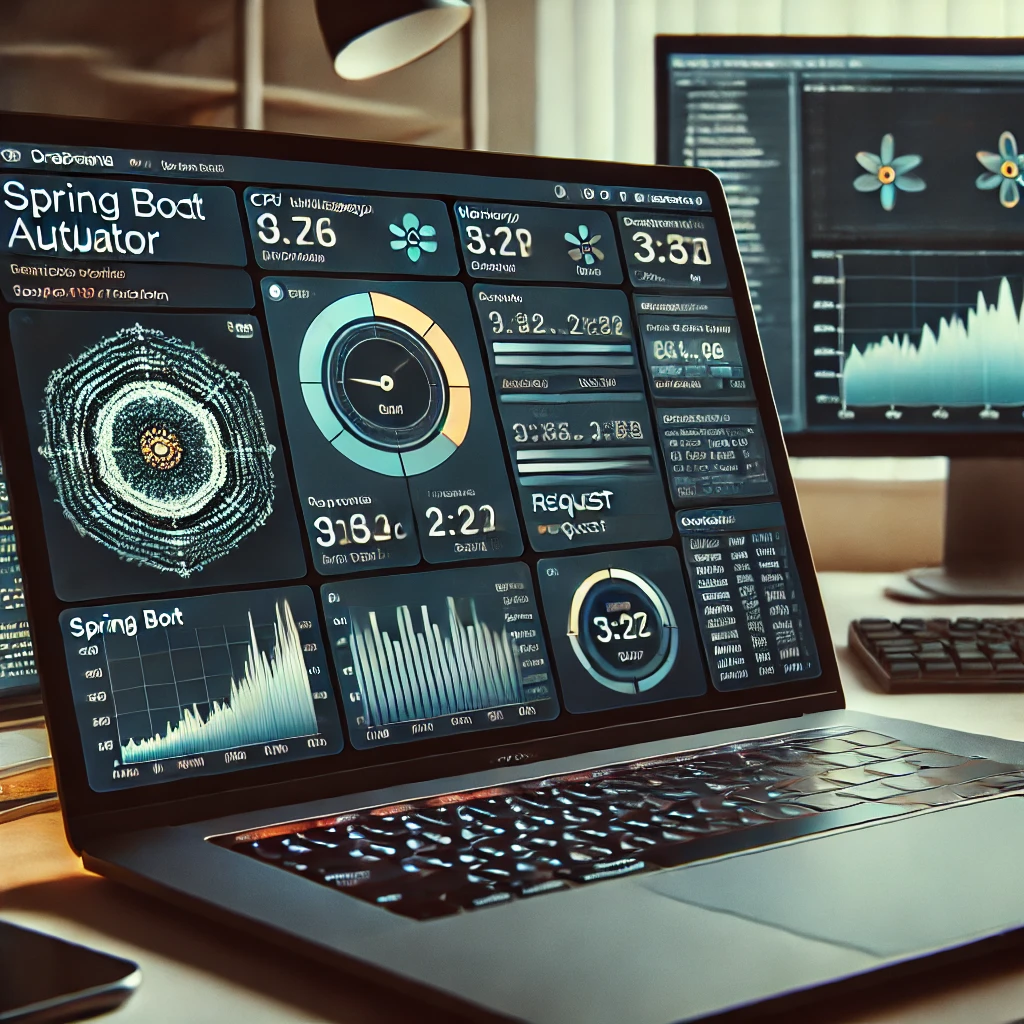
6. Integrate Actuator into monitoring systems
Spring Boot Actuator can be easily integrated into monitoring systems such as Prometheus and Grafana.
Using Micrometer and Prometheus
Spring Boot uses Micrometer to provide metrics. The Prometheus integration can be activated with the following dependency:
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
</dependency>
A new endpoint then becomes available:
http://localhost:8080/actuator/prometheus
This can be queried from a Prometheus server and visualized in Grafana.
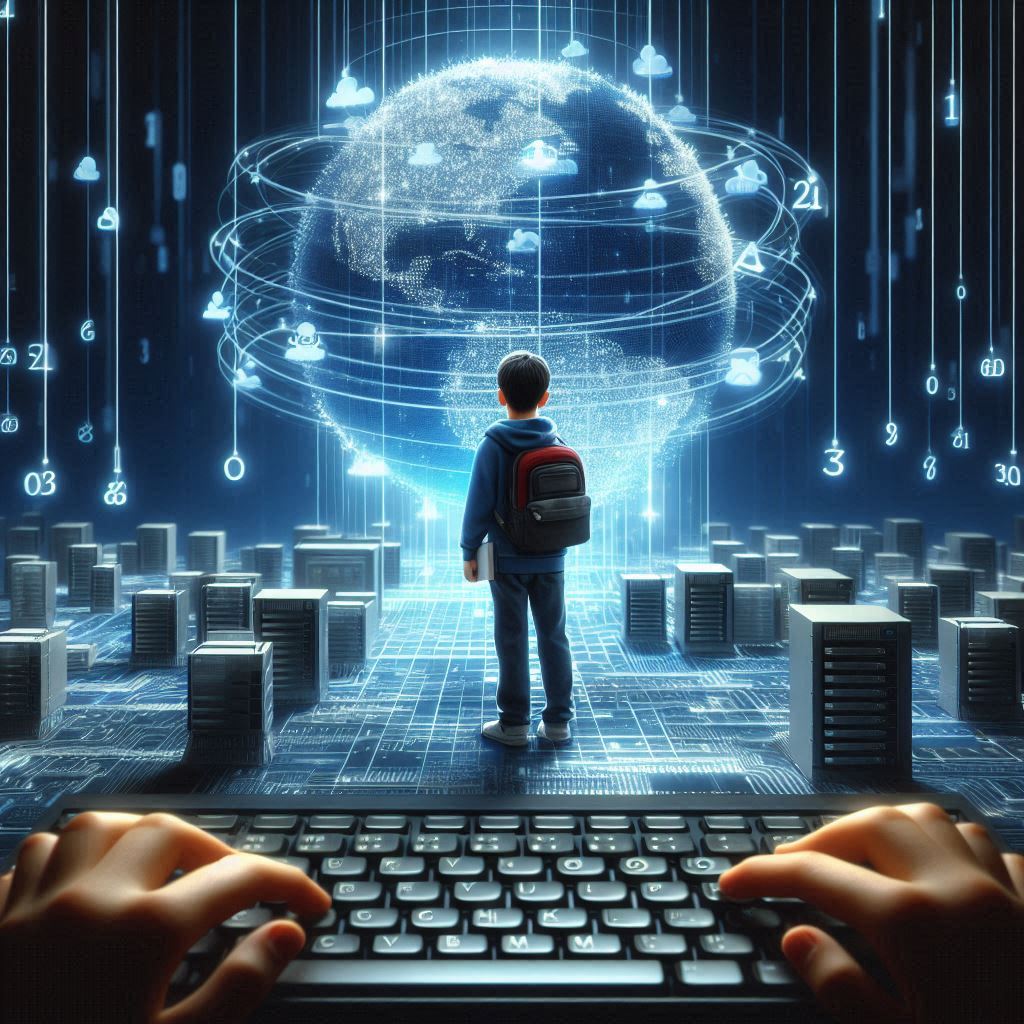
7. Create your own actuator endpoints
Spring Boot allows you to define your own actuator endpoints if special information is to be provided.
Example: Custom Actuator Endpoint
@Component
@Endpoint(id = "custom-info")
public class CustomInfoEndpoint {
@ReadOperation
public Map<String, String> customInfo() {
return Map.of("appVersion", "1.0.0", "author", "John Doe");
}
}
The new endpoint can then be reached under /actuator/custom-info.
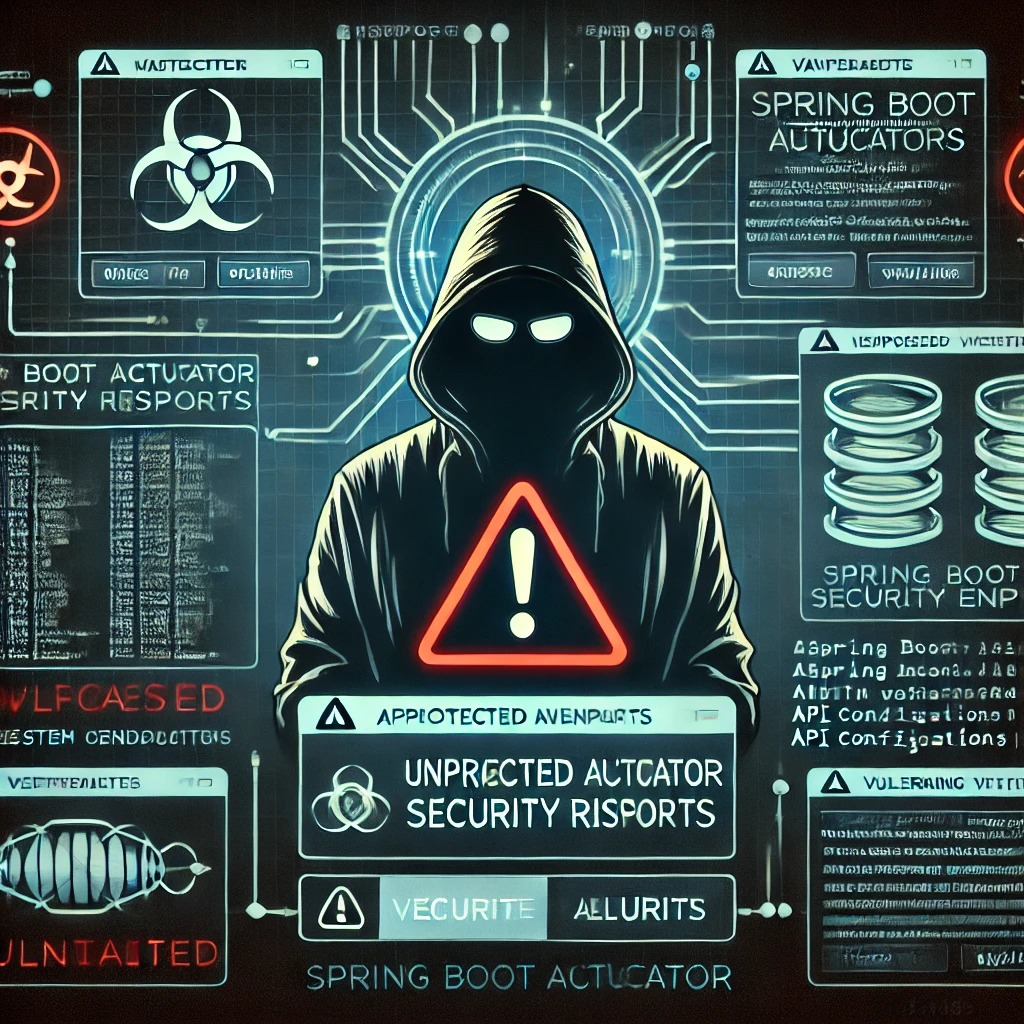
8. Dangers due to unprotected actuator endpoints
Spring Boot Actuator offers many useful functions for monitoring and managing an application. However, if these endpoints are not properly secured, they can be exploited by attackers. Here are some of the most critical endpoints and possible attacks:
1. /env (up to Spring Boot 2.x) or /actuator/configprops
- Risk: Displays environment variables and configuration values, including API keys, passwords and other sensitive data.
- Attack: Attackers can read this data and use it for further attacks, e.g. to log into external services.
2. /heapdump
- Risk: Allows the download of a memory image of the application, which may contain session IDs, tokens or passwords.
- Attack: An attacker can analyze the dump and extract internal data or access data.
3. /loggers
- Risk: Allows the log level to be changed at runtime.
- Attack: An attacker could set the logs to DEBUG or TRACE and thus obtain detailed application information.
4. /mappings
- Risk: Shows all registered API endpoints of the application.
- Attack: By scanning this list, an attacker can find and target hidden or insecure endpoints.
5. /beans
- Risk: Lists all registered beans and their dependencies.
- Attack: Gives attackers valuable information about the internal architecture of the application, which they can use for attacks such as dependency injection or exploits.
6. /threaddump
- Risk: Returns a list of all running threads of the JVM.
- Attack: Can be used to analyze internal processes or intercept running transactions.
7. /shutdown (up to Spring Boot 2.x, disabled by default)
- Risk: Allows the application to be shut down via HTTP request.
- Attack: If enabled, an attacker could simply send a request and shut down the application.
Security measures against actuator attacks
To prevent Actuator endpoints from being exploited by unauthorized persons, the following measures should be implemented:
Make endpoints accessible only to administrators
- Use Basic Auth or OAuth2 for access.
- Only allow certain users to access sensitive endpoints.
Restrict endpoints to internal networks
- Only allow access via localhost or a VPN.
- Set firewall rules to block unauthorized requests.
Deactivate endpoints that are not required
- In the application.properties or application.yml:
- Â
management.endpoints.web.exposure.exclude=env,heapdump,loggers,shutdown
- Alternativ explizit nur benötigte Endpunkte freigeben:
management.endpoints.web.exposure.include=health,info
Activate Spring Security for Actuator endpoints
- Protection via Spring Security to allow only authenticated users.
- Example of a security configuration:
@Configuration
public class ActuatorSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/actuator/health", "/actuator/info").permitAll()
.antMatchers("/actuator/**").hasRole("ADMIN")
.and()
.httpBasic();
}
}
Conclusion
Spring Boot Actuator is a powerful tool for monitoring and analyzing applications. Through the clever configuration and use of security measures, it can offer enormous benefits to both developers and DevOps teams. With integration into monitoring tools such as Prometheus and Grafana, meaningful dashboards can be created that make application operation much easier.