A Spring Boot application without logging and monitoring is like driving a car without a speedometer and rear-view mirror – you can’t see what’s happening until it’s too late. A good logging and monitoring strategy not only helps with troubleshooting, but also with security and performance optimization. In this article, we take a practical look at logging and monitoring in Spring Boot, including code examples, proven tools and best practices.
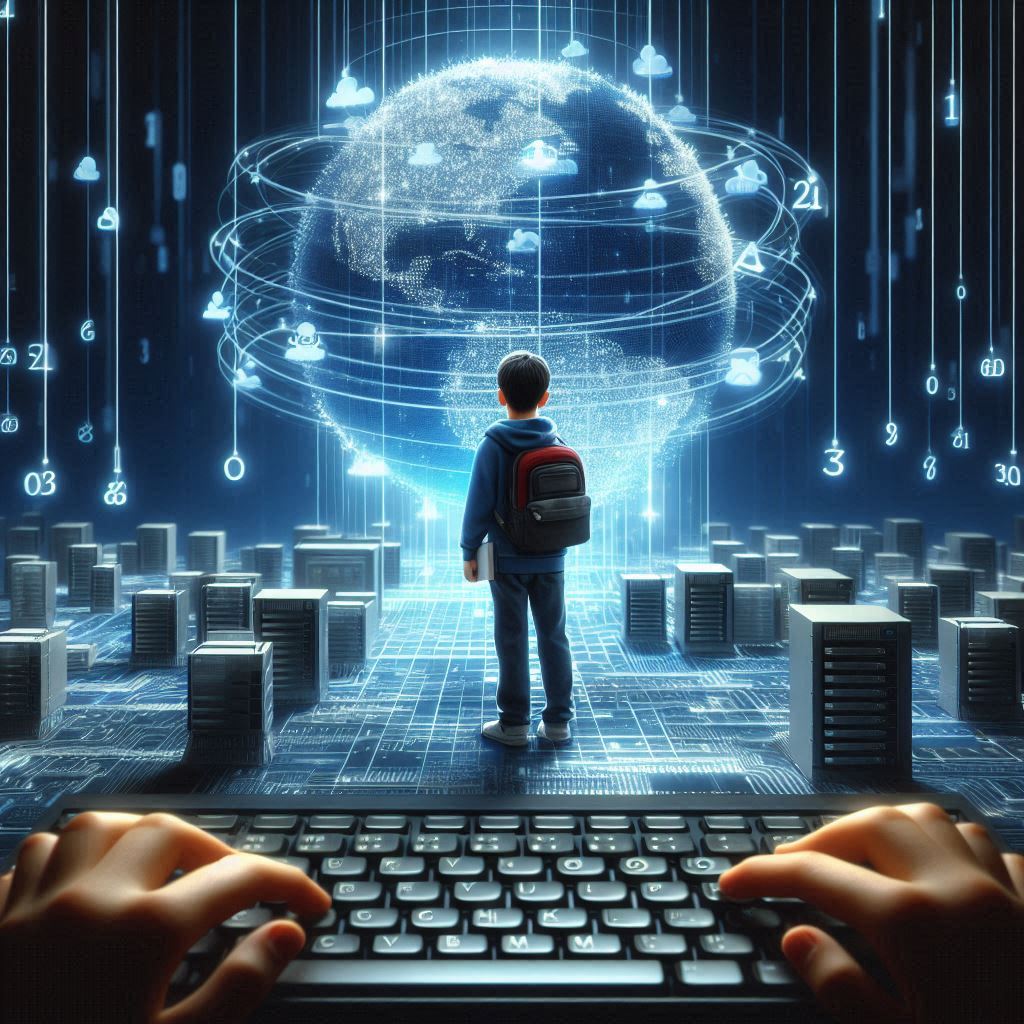
1. Logging in Spring Boot: The Basics
Spring Boot relies on SLF4J with Logback as the logging framework by default. This is a solid basis that can be expanded as required.
1.1 Configuring Logback
Spring Boot comes with a default configuration, but we can customize it by creating a logback-spring.xml file in the resources directory:
<configuration>
<appender name="CONSOLE" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<root level="INFO">
<appender-ref ref="CONSOLE"/>
</root>
</configuration>
This ensures that logs end up properly formatted in the console.
1.2 Use logging in the application
Now we can write logs in our services:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Service;
@Service
public class UserService {
private static final Logger logger = LoggerFactory.getLogger(UserService.class);
public void processUser(String userId) {
logger.info("Starte Verarbeitung für Benutzer: {}", userId);
try {
// Simulierte Verarbeitung
Thread.sleep(1000);
} catch (InterruptedException e) {
logger.error("Fehler bei der Verarbeitung von Benutzer {}: {}", userId, e.getMessage());
}
}
}
1.3 Dynamically adjusting the log level
Sometimes you want to change the log level without changing the code. This is easily done via the application.properties:
logging.level.org.springframework=DEBUG
logging.level.com.example=INFO
Or at runtime via actuator endpoint:
curl -X POST localhost:8080/actuator/loggers/com.example -H "Content-Type: application/json" -d '{"configuredLevel": "DEBUG"}'
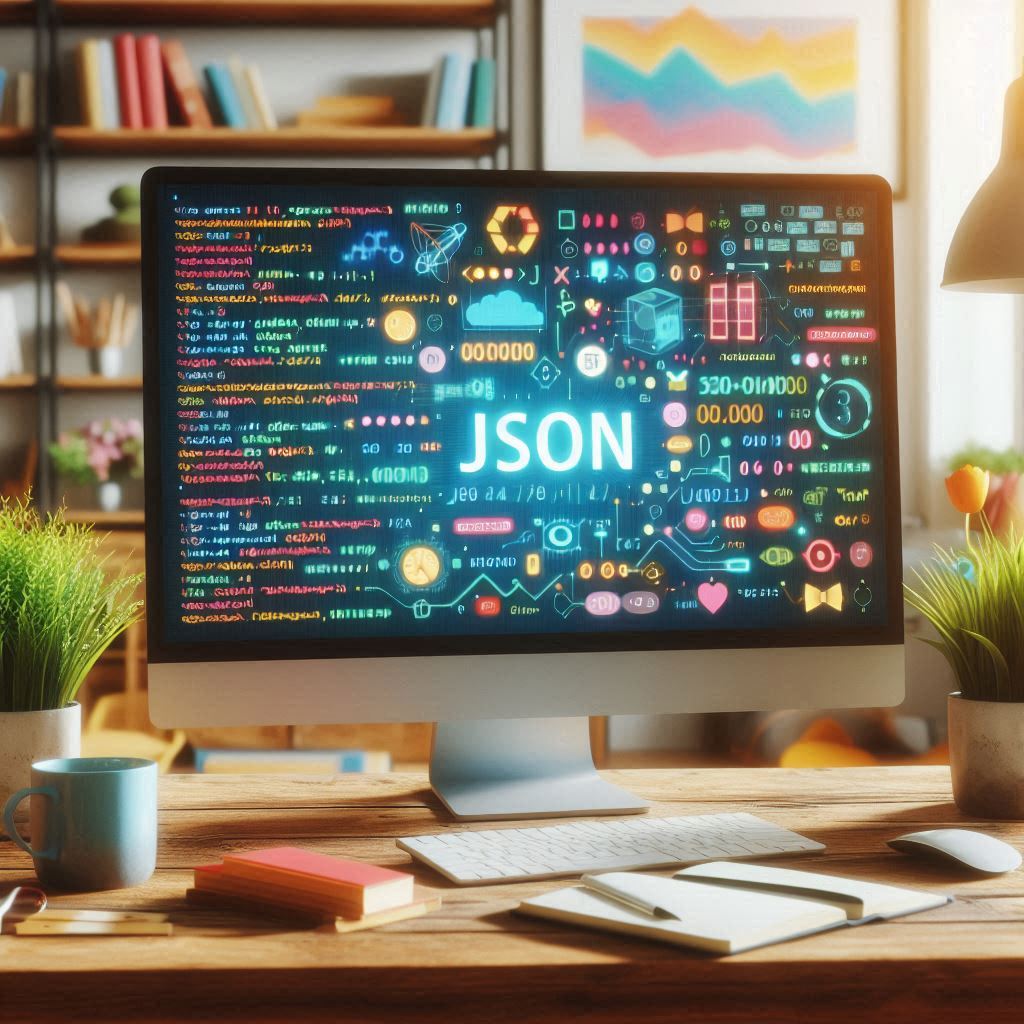
2. Structured logs with JSON
Normal text logs are difficult to analyze. A better alternative is logging in JSON format, which is particularly useful for centralized logging systems such as Elasticsearch, Logstash and Kibana (ELK).
2.1. JSON-Logging with Logstash-Encoder
Extend the pom.xml with the Logstash encoder dependency. You can find the latest version here:
<dependency>
<groupId>net.logstash.logback</groupId>
<artifactId>logstash-logback-encoder</artifactId>
<version>7.0</version>
</dependency>
And adjust the logback-spring.xml:
<appender name="LOGSTASH" class="ch.qos.logback.core.rolling.RollingFileAppender">
<file>logs/app.log</file>
<encoder class="net.logstash.logback.encoder.LoggingEventCompositeJsonEncoder"/>
</appender>
<root level="INFO">
<appender-ref ref="LOGSTASH"/>
</root>
Now the logs are saved as JSON – ideal for integration with centralized logging systems.
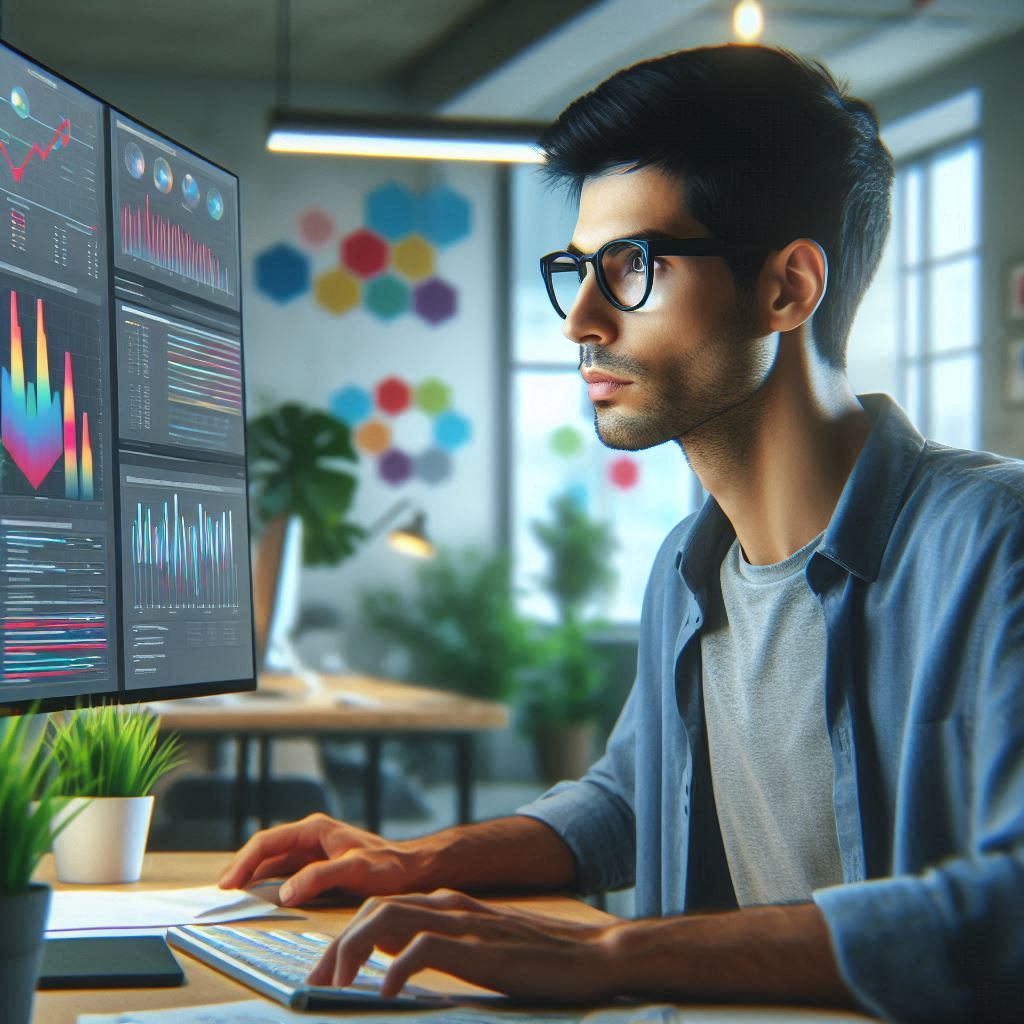
3. Monitoring in Spring Boot: The Essentials
Logging shows us what has already happened – monitoring helps us to recognize problems in real time. Spring Boot offers a powerful monitoring solution with Spring Boot Actuator.
3.1 Why not all actuators should be released
Spring Boot Actuator offers a variety of endpoints that provide valuable information about the application. However, not all endpoints should be publicly accessible as they may contain sensitive data.
Risks with unprotected Actuator endpoints:
- Information Disclosure: Attackers can read internal details such as environment variables, metrics or configuration values.
- Manipulation: Some endpoints (e.g. /actuator/loggers) allow log levels to be changed, which can be used to conceal attack traces.
- Denial of Service (DoS): The /actuator/heapdump endpoint can deliver large memory dumps, which can lead to memory problems if used uncontrolled.
Best practices for secure Actuator usage:
- Enable only necessary endpoints (management.endpoints.web.exposure.include=health,info,metrics)
- Secure Actuator endpoints with Basic Auth or OAuth 2.0
- Restrict access to internal networks
3.2 Using Prometheus for metrics
A logging system is not enough – we also need metrics. Prometheus is a popular choice here.
Install the Micrometer-Prometheus-Dependency:
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
</dependency>
Add in application.properties:
management.metrics.export.prometheus.enabled=true
management.endpoints.web.exposure.include=prometheus
Now http://localhost:8080/actuator/prometheus provides all the metrics that Prometheus can process.
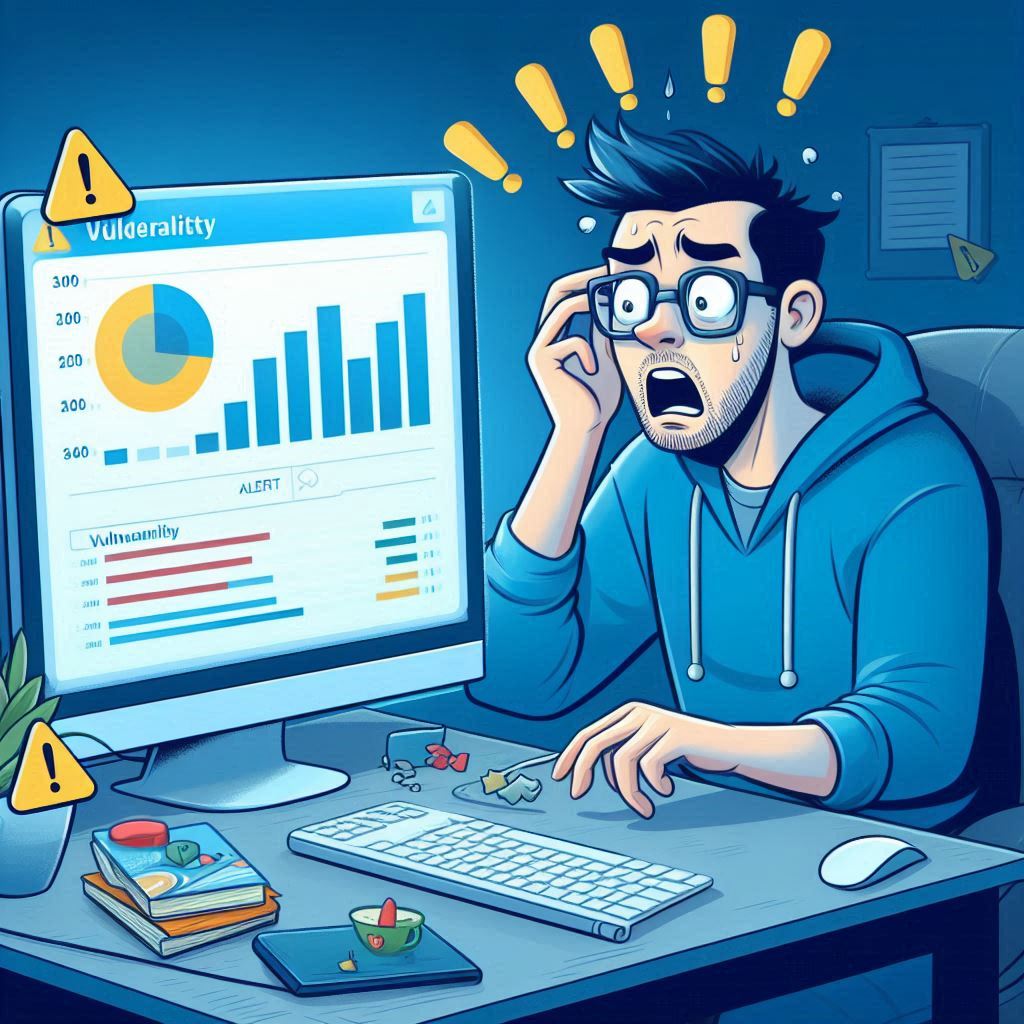
4. Avoid Log4j vulnerability
The Log4j vulnerability (CVE-2021-44228) allows remote code execution (RCE) through manipulated log entries. Attackers can inject and execute malicious code. If you are more interested in the topic of IT security, take a look at our blog.
4.1 Impact of the Log4j vulnerability
- Remote Code Execution (RCE): Attackers can execute malicious code.
- Data leaks: Sensitive information can be exfiltrated from the logs.
- System takeover: Attackers may gain complete control over the system.
4.2. Security Controls
- Change Spring Boot to Logback or SLF4J: Replace Log4j with safer alternatives.
- Use the latest Log4j version: If Log4j is required, use at least version 2.17.1.
- Deactivate JNDI lookup: set log4j2.formatMsgNoLookups=true in the system variables.
Conclusion
Logging and monitoring are essential for a stable Spring Boot application. With Logback, Actuator, Prometheus and ELK, we have powerful tools at hand. If you combine logs and metrics wisely, you can find errors faster, detect attacks and optimize performance.
💡 Tip: Automate your logging and monitoring with Docker and Kubernetes for scalable solutions!
You should always make sure that your applications are secure. A vulnerability like the Log4j vulnerability can occur at any time. Therefore, always keep your dependencies and the Spring Boot version up to date. We recommend that you get professional support, e.g. by carrying out a penetration test or seeking IT security advice. Take a look at our marketplace for information, IT and cyber security and find a suitable provider who can help you.