There are many security vulnerabilities in web applications, but some only become apparent when they are actively exploited. Open redirects are just such a case. This vulnerability is often underestimated, but can have massive consequences. In this article, I’ll explain what open redirects are, how they are exploited in real-life scenarios and how you can protect your web applications against them.
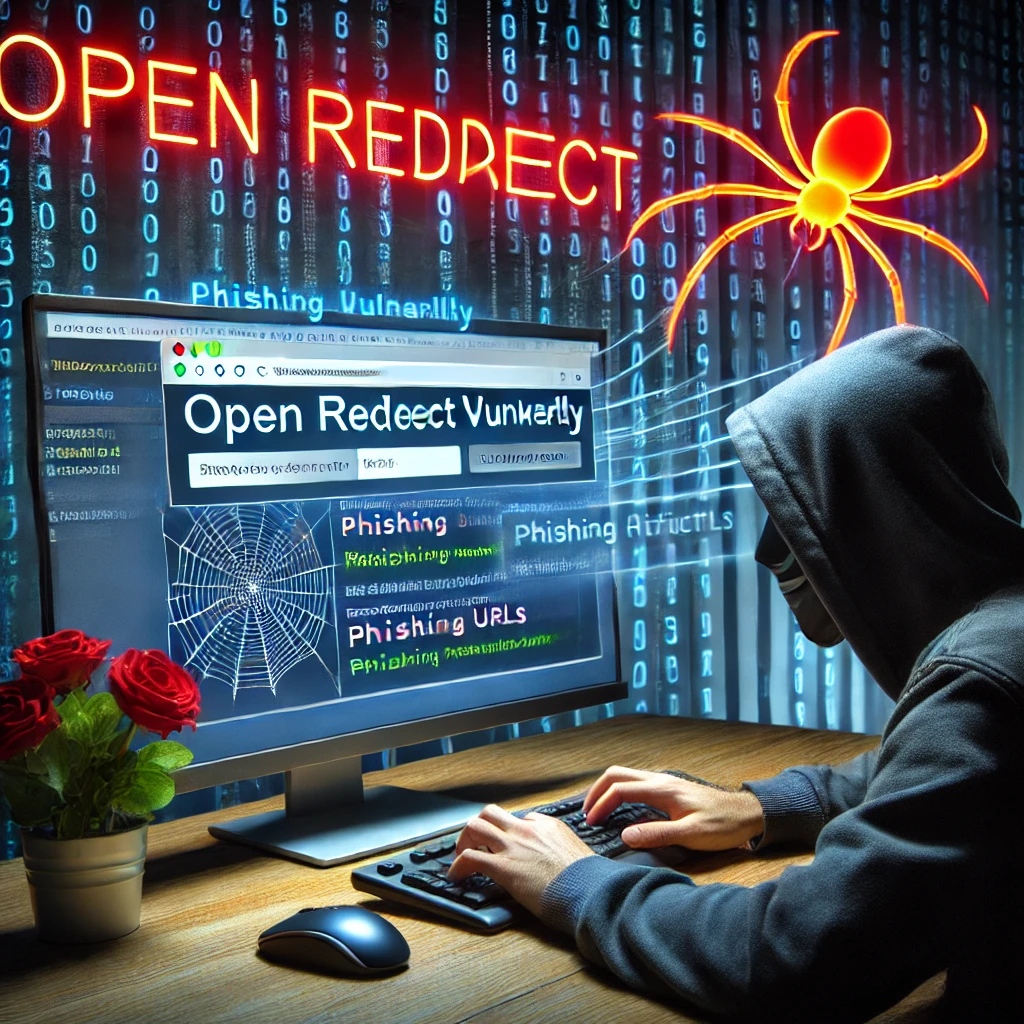
What is an open redirect?
An open redirect occurs when an application allows users to be redirected to an external URL without checking whether the target URL is trustworthy. This often happens because the target URL is passed as a parameter in the request.
Example:
<a href="https://example.com/redirect?url=https://evilpage.com">Click Here</a>
If the application does not validate the URL, the user is redirected to a malicious page. This technique is often used for phishing attacks or session hijacking.
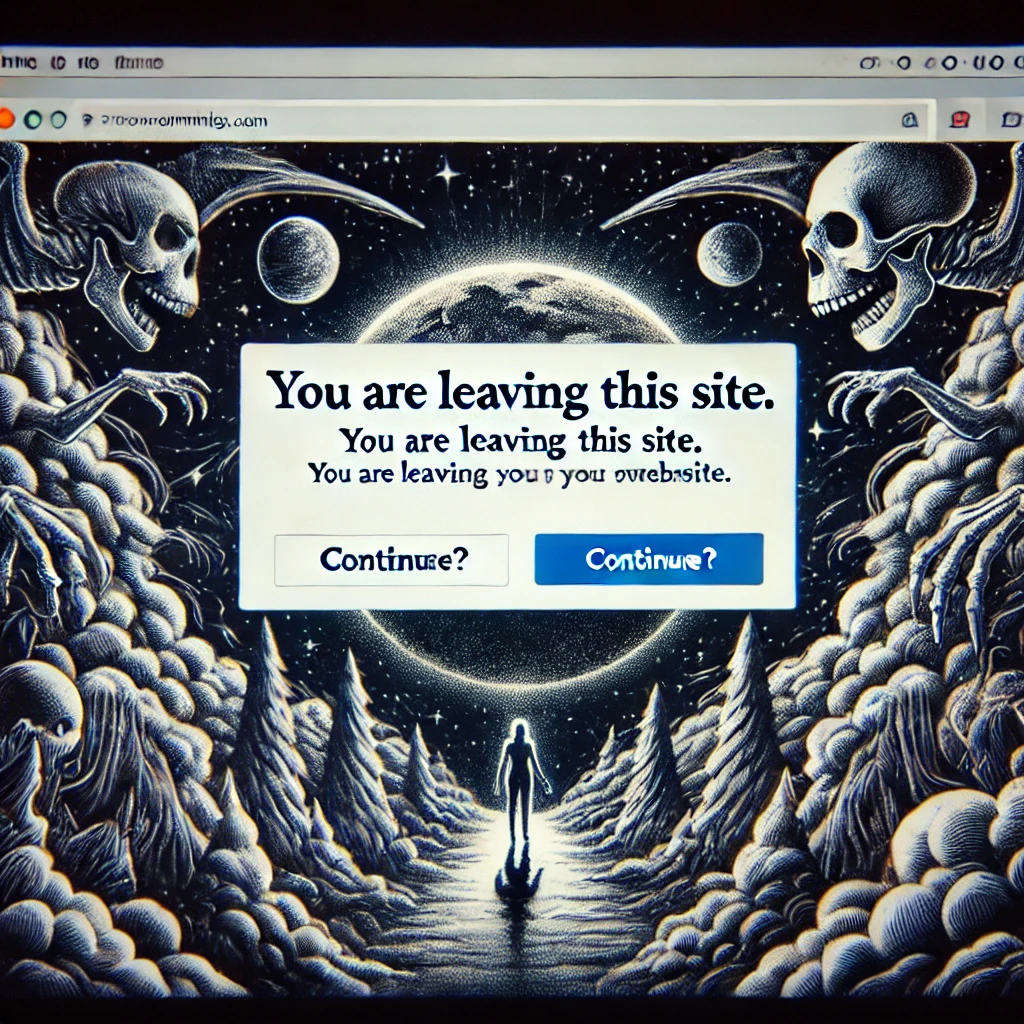
Practical attack scenarios
1. Phishing with legitimate domains
An attacker can use a legitimate-looking URL to redirect the victim to a fraudulent page. Example:
https://trusted-bank.com/login?redirect=https://hacker-site.com/fake-login
Here the user thinks he is logging in to his bank, but ends up on a fake login page.
2. Open Redirect as part of an XSS chain
If a website is vulnerable to open redirects, an attacker can use this in combination with cross-site scripting (XSS) to execute malicious scripts.
3. Malware-Downloads
Open redirects can lead users unnoticed to a page that automatically downloads malware or contains exploits.
4. Open Redirect and OAuth-Bypassing
Some web applications use open redirects in authentication processes. For example, an OAuth implementation could contain the following redirect:
https://example.com/oauth?redirect=https://website.com/dashboard
If attackers can manipulate the redirect URL, this could result in users being redirected to a fake page after authentication, where their session information is harvested.
5. Combination with CSRF (Cross-Site Request Forgery)
In combination with CSRF attacks, an open redirect can be used to direct users unnoticed to a malicious page while they simultaneously perform a malicious action on another page.
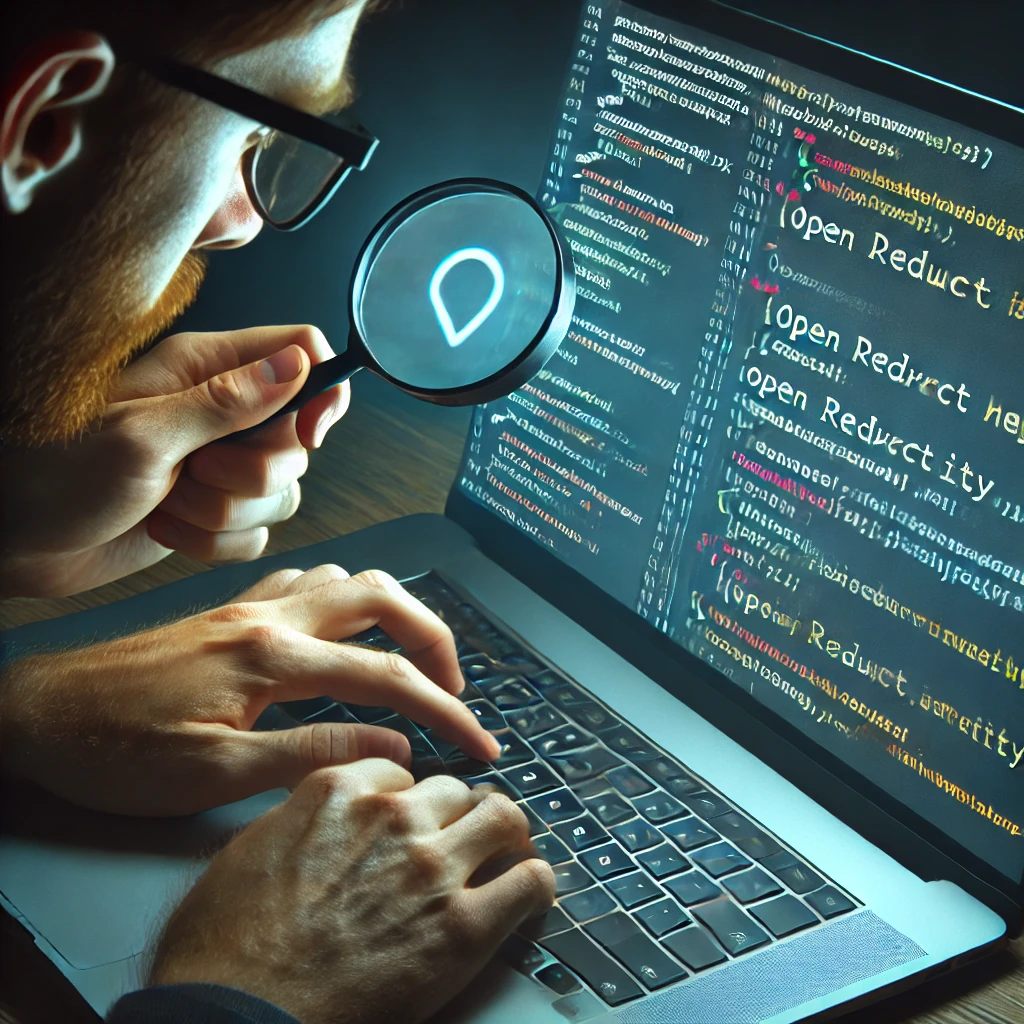
How can you protect yourself from open redirects?
1. Whitelisting of forwarding destinations
Only allow known, trusted domains as redirect destinations. Example in PHP:
$allowed_hosts = ['example.com', 'mytrusteddomain.com'];
$parsed_url = parse_url($_GET['url']);
if (!in_array($parsed_url['host'], $allowed_hosts)) {
die("Ungültige Weiterleitung");
}
header("Location: " . $_GET['url']);
2. Using relative URLs
If internal forwarding is required, only a relative URL should be used:
header("Location: /dashboard");
3. User warnings
If external forwarding is unavoidable, the user should be warned:
echo "You are getting forwarded to: " . htmlspecialchars($_GET['url']);
4. Protection in various programming languages
Java
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Arrays;
import java.util.List;
public class OpenRedirectProtection {
private static final List<String> ALLOWED_DOMAINS = Arrays.asList("example.com", "trusted.com");
public static boolean isValidRedirect(String url) {
try {
URI uri = new URI(url);
return ALLOWED_DOMAINS.contains(uri.getHost());
} catch (URISyntaxException e) {
return false;
}
}
public static void main(String[] args) {
String userUrl = "https://example.com/dashboard";
if (isValidRedirect(userUrl)) {
System.out.println("Weiterleitung erlaubt");
} else {
System.out.println("Ungültige Weiterleitung blockiert");
}
}
}
JavaScript
const allowedDomains = ['example.com', 'trusted.com'];
function isValidRedirect(url) {
try {
const parsedUrl = new URL(url);
return allowedDomains.includes(parsedUrl.hostname);
} catch (e) {
return false;
}
}
const userRedirect = 'https://malicious-site.com';
if (isValidRedirect(userRedirect)) {
window.location.href = userRedirect;
} else {
alert('Ungültige Weiterleitung blockiert');
}
PHP
function isValidRedirect($url) {
$allowed_hosts = ['example.com', 'trusted.com'];
$parsed_url = parse_url($url);
return isset($parsed_url['host']) && in_array($parsed_url['host'], $allowed_hosts);
}
$user_url = $_GET['url'] ?? '';
if (isValidRedirect($user_url)) {
header("Location: $user_url");
exit;
} else {
die("Ungültige Weiterleitung blockiert");
}
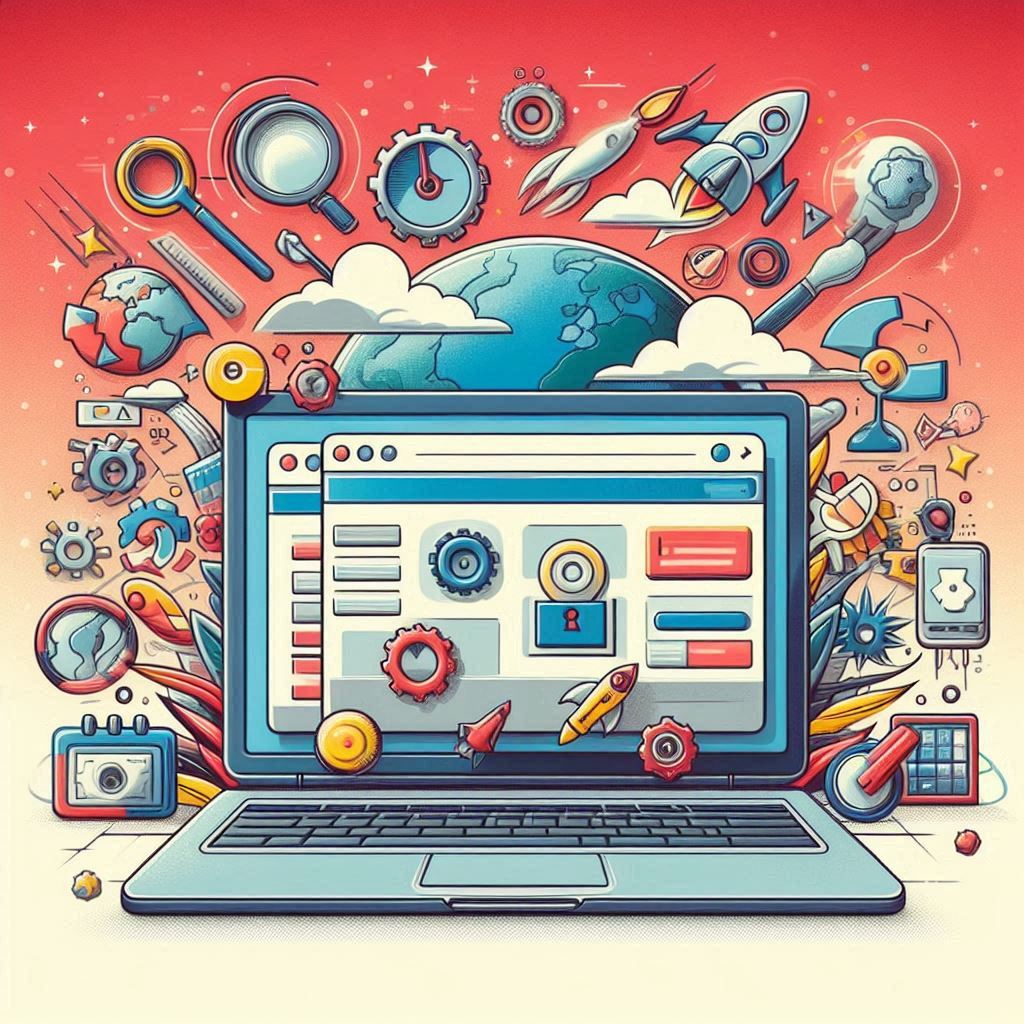
Which tools help to recognize open redirects?
There are various tools that can help to find vulnerabilities such as open redirects in a web application:
1. Burp Suite
Burp Suite is a powerful tool for security testing. It can intercept and manipulate HTTP requests to detect vulnerabilities such as open redirects.
2. OWASP ZAP
OWASP Zed Attack Proxy (ZAP) is an open source security scanner that automatically tests for open redirects and documents them in reports.
3. Google Dorks
Targeted search queries (Google Dorks) can be used to check whether suspicious redirects are publicly indexed.
Example:
site:example.com inurl:redirect=
4. Nikto
Nikto is a web scanner that can detect known security vulnerabilities, including insecure redirects.
5. Manual testing with DevTools
Open redirects can often also be detected by manual testing in the browser’s developer tools by manipulating redirect URLs and checking the application’s reactions.
Conclusion
Open redirects are an underestimated but dangerous vulnerability. You can effectively secure your application with whitelisting, relative URLs, user warnings, logging and monitoring. If you are a developer, check your application for this vulnerability with the tools mentioned above before an attacker does!